# 18.5:获取设备尺寸
读者在 App 开发过程中可能会有用到屏幕尺寸的场景,比如需要知道屏幕的宽高,状态栏和导航栏的高度等做些计算,本节笔者简单演示一下如何在 OpenHarmony 上获取到这些尺寸信息。
# 18.5.1:获取屏幕宽高
ArkUI 开发框架在 @ohos.display
模块里增加了获取屏幕尺寸的方法 getDefaultDisplay(),通过该方法可以获取到屏幕尺寸信息,代码如下:
private getScreenSize() {
display.getDefaultDisplay().then((defaultDisplay) => {
this.screenWidth = defaultDisplay.width
this.screenHeight = defaultDisplay.height
this.allMsg = JSON.stringify(defaultDisplay)
}).catch((error) => {
this.screenWidth = -1
this.screenHeight = -1
this.allMsg = JSON.stringify(error)
})
}
1
2
3
4
5
6
7
8
9
10
11
2
3
4
5
6
7
8
9
10
11
# 18.5.2:获取状态栏高度
获取状态栏高度采用的是 Window
对象的 getAvoidArea() 方法,该方法表示的是获取当前窗口上系统使用的特殊区域,这些特殊区域三方应用无法占用,比如状态栏和导航栏区域,代码如下:
// FA 模型
private getStatusBarHeight() {
window.getTopWindow().then(topWindow => {
topWindow.getAvoidArea(window.AvoidAreaType.TYPE_SYSTEM).then(avoidArea => {
this.statusBarHeight = avoidArea.topRect.height
})
})
}
// Stage 模型
private getStatusBarHeight() {
var context = getContext(this) as context.Context
window.getLastWindow(context).then((lastWindow) => {
lastWindow.getAvoidArea(window.AvoidAreaType.TYPE_SYSTEM).then(avoidArea => {
this.statusBarHeight = avoidArea.topRect.height
})
}).catch((error) => {
console.log("getLastWindow error: " + JSON.stringify(error))
})
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
# 18.5.3:获取导航栏高度
获取状态栏高度采用的是 Window
对象的 getAvoidArea() 方法,该方法表示的是获取当前窗口上系统使用的特殊区域,这些特殊区域三方应用无法占用,比如状态栏和导航栏区域,代码如下:
// FA 模型
private getNavigationBarHeight() {
window.getTopWindow().then((topWindow) => {
topWindow.getAvoidArea(window.AvoidAreaType.TYPE_SYSTEM).then((avoidArea) => {
this.navigationBarHeight = avoidArea.bottomRect.height
})
})
}
// Stage 模型
private getNavigationBarHeight() {
var context = getContext(this) as context.Context
window.getLastWindow(context).then((lastWindow) => {
lastWindow.getAvoidArea(window.AvoidAreaType.TYPE_SYSTEM).then(avoidArea => {
this.statusBarHeight = avoidArea.bottomRect.height
})
}).catch((error) => {
console.log("getLastWindow error: " + JSON.stringify(error))
})
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
# 18.5.4:完整样例演示
import display from '@ohos.display';
import window from '@ohos.window';
@Entry @Component struct ArkUIClubTest {
@State screenWidth: number = 0
@State screenHeight: number = 0
@State statusBarHeight: number = 0
@State navigationBarHeight: number = 0
@State allMsg: string = ""
@State sysMsg: string = ""
build() {
Column({space: 10}) {
Text("屏幕宽度:" + this.screenWidth + " px")
.fontSize(22)
Text("屏幕高度:" + this.screenHeight + " px")
.fontSize(22)
Text(this.allMsg)
.fontSize(18)
Button("获取屏幕尺寸")
.onClick(() => {
this.getScreenSize()
})
Text("状态栏高度:" + this.statusBarHeight + " px")
.fontSize(22)
Text("导航栏高度:" + this.navigationBarHeight + " px")
.fontSize(22)
Text(this.sysMsg)
.fontSize(18)
Button("获取状态栏和导航栏高度")
.onClick(() => {
this.getSystemBarHeight()
})
}
.width("100%")
.height("100%")
.padding(10)
}
// FA 模型获取 Window
private getSystemBarHeight() {
window.getTopWindow().then((topWindow) => {
this.getSystemBarHeightInternal(topWindow)
}).catch((error) => {
this.statusBarHeight = -1
this.navigationBarHeight = -1
this.sysMsg = JSON.stringify(error)
})
}
// Stage 模型获取 Window
/**
private getSystemBarHeight() {
var context = getContext(this) as context.Context
window.getLastWindow(context).then((lastWindow) => {
this.getSystemBarHeightInternal(lastWindow)
}).catch((error) => {
this.statusBarHeight = -1
this.navigationBarHeight = -1
this.sysMsg = JSON.stringify(error)
})
}
*/
private getSystemBarHeightInternal(mainWindow: window.Window) {
mainWindow.getAvoidArea(window.AvoidAreaType.TYPE_SYSTEM).then((avoidArea) => {
this.statusBarHeight = avoidArea.topRect.height
this.navigationBarHeight = avoidArea.bottomRect.height
}).catch((error) => {
this.statusBarHeight = -1
this.navigationBarHeight = -1
this.sysMsg = JSON.stringify(error)
})
}
private getScreenSize() {
display.getDefaultDisplay().then((defaultDisplay) => {
this.screenWidth = defaultDisplay.width
this.screenHeight = defaultDisplay.height
this.allMsg = JSON.stringify(defaultDisplay)
}).catch((error) => {
this.screenWidth = -1
this.screenHeight = -1
this.allMsg = JSON.stringify(error)
})
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
样例运行结果如下图所示:
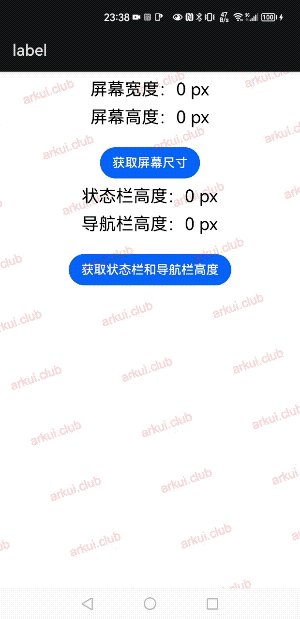
# 18.5.5:小结
本节向读者演示了如何获取屏幕宽高、状态栏高度和导航栏高度的样例供读者参考,另外需要注意的是获取状态栏和导航栏高度的写法在 FA 模型和 Stage 模型下是不一样的,最后也非常欢迎小伙伴能给本网站提供更多的开发样例。
← 18.4:横竖屏切换 18.6:页面置灰 →
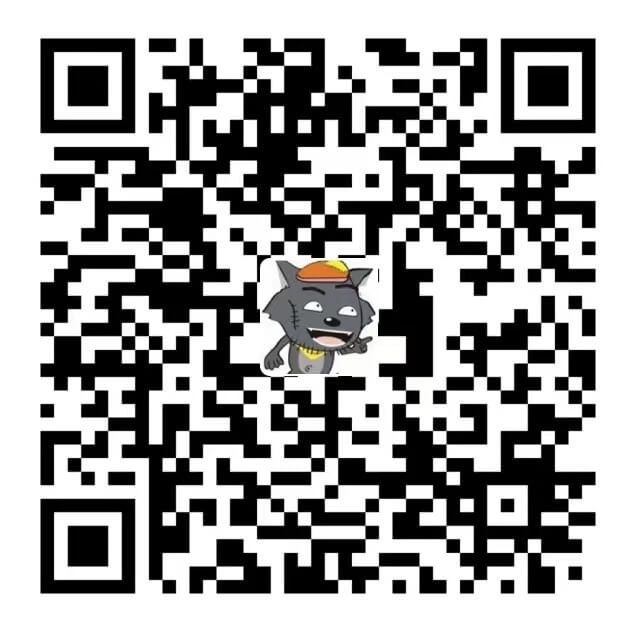
请作者喝杯咖啡
©arkui.club版权所有,禁止私自转发、克隆网站。