# 5.7:角标组件(Badge)
ArkUI 开发框架提供了 Badge 容器组件,它用来标记提示信息的容器组件,最常用的场景比如 Launcher
桌面上的消息提示等,本节笔者简单介绍一下 Badge 的使用。
# 5.7.1:Badge 定义介绍
interface BadgeInterface {
(value: BadgeParamWithNumber): BadgeAttribute;
(value: BadgeParamWithString): BadgeAttribute;
}
declare interface BadgeParam {
position?: BadgePosition;
style: BadgeStyle;
}
declare interface BadgeParamWithNumber extends BadgeParam {
count: number;
maxCount?: number;
}
declare interface BadgeParamWithString extends BadgeParam {
value: string;
}
declare interface BadgeParam {
position?: BadgePosition;
style: BadgeStyle;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
Badge 的构造方法允许接收 BadgeParamWithNumber
和 BadgeParamWithString
两种类型的参数,它们都继承自 BadgeParam
,BadgeParam
参数说明如下:
position:设置 badge 的显示位置,
BadgePosition
提供了以下 3 种位置:- Right: badge 显示在右侧纵向居中。
- RightTop(默认值): badge 显示在右上角。
- Left: badge 显示在左侧纵向居中。
style:设置 badge 的显示样式,
BadgeStyle
样式参数说明如下:- color:设置 badge 的文本颜色,默认为白色。
- fontSize:设置 badge 的文本字体大小,默认为 10 vp。
- badgeSize:设置 badge 的显示大小。
- badgeColor:设置 badge 的背景颜色,默认为红色。
简单样例如下所示:
@Entry @Component struct ComponentTest { build() { Column() { Row({space: 20}) { Badge({ value: ' ', position: BadgePosition.Left, // 设置 badge 居左显示 style: { badgeSize: 10, badgeColor: Color.Red } // 设置 badge 的显示样式 }) { Text("Badge") .size({ width: 100, height: 50 }) .fontSize(20) .backgroundColor("#aabbcc") } .size({ width: 100, height: 50 }) Badge({ value: ' ', position: BadgePosition.Right, // 设置 badge 居右显示 style: { badgeSize: 10, badgeColor: Color.Red } // 设置 badge 的显示样式 }) { Text("Badge") .size({ width: 100, height: 50 }) .fontSize(20) .backgroundColor("#aabbcc") } .size({ width: 100, height: 50 }) Badge({ value: ' ', position: BadgePosition.RightTop, // 设置 badge 居右上角显示 style: { badgeSize: 10, badgeColor: Color.Red } // 设置 badge 的显示样式 }) { Text("Badge") .size({ width: 100, height: 50 }) .fontSize(20) .backgroundColor("#aabbcc") } .size({ width: 100, height: 50 }) } } .width("100%") .height("100%") .padding(20) } }
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47@Entry @Component class MyView { func build() { Column(10) { Row(20) { Badge(BadgeParams( count: 1, style: BadgeStyle(color: Color(0xFFFFFF), fontSize: 16, badgeSize: 20, badgeColor: Color.RED), position: BadgePosition.Left, maxCount: 99) ) { Text("Badge") .size(width: 100, height: 50) .fontSize(20) .backgroundColor(0xaabbcc) } .size(width: 100, height: 50) Badge(BadgeParams( count: 1, style: BadgeStyle(color: Color(0xFFFFFF), fontSize: 16, badgeSize: 20, badgeColor: Color.RED), position: BadgePosition.Right, maxCount: 99) ) { Text("Badge") .size(width: 100, height: 50) .fontSize(20) .backgroundColor(0xaabbcc) } .size(width: 100, height: 50) Badge(BadgeParams( count: 1, style: BadgeStyle(color: Color(0xFFFFFF), fontSize: 16, badgeSize: 20, badgeColor: Color.RED), position: BadgePosition.RightTop, maxCount: 99) ) { Text("Badge") .size(width: 100, height: 50) .fontSize(20) .backgroundColor(0xaabbcc) } .size(width: 100, height: 50) } } .padding(10) .width(100.percent) .height(100.percent) } }
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49样例运行结果如下图所示:
BadgeParamWithNumber
可以根据数字创建提醒组件,各参数说明如下:
- count:设置提醒消息数。
- maxCount:设置提醒消息的最大数,超过最大消息时仅显示 maxCount+。
简单样例如下所示:
@Entry @Component struct ComponentTest {
build() {
Column() {
Row({space: 20}) {
Badge({
count: 10, // 设置 badge 显示的数量
maxCount: 100, // 设置 badge 显示的最大数量
position: BadgePosition.RightTop,// 设置 badge 显示在右上角
style: {badgeColor: Color.Red} // 设置 badge 的显示样式
}) {
Text("Badge")
.size({width: 100, height: 50})
.fontSize(20)
.backgroundColor("#aabbcc")
}
.size({width: 100, height: 50})
Badge({
count: 110, // 设置 badge 显示的数量
maxCount: 99, // 设置 badge 显示的最大数量
position: BadgePosition.RightTop,// 设置 badge 显示在右上角
style: {badgeColor: Color.Red} // 设置 badge 的显示样式
}) {
Text("Badge")
.size({width: 100, height: 50})
.fontSize(20)
.backgroundColor("#aabbcc")
}
.size({width: 100, height: 50})
}
}
.width("100%")
.height("100%")
.padding(20)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
@Entry @Component class MyView {
func build() {
Column(10) {
Row(20) {
Badge(BadgeParams(
count: 10,
style: BadgeStyle(color: Color(0xFFFFFF), fontSize: 16, badgeSize: 20, badgeColor: Color.RED),
position: BadgePosition.RightTop,
maxCount: 99)
) {
Text("Badge")
.size(width: 100, height: 50)
.fontSize(20)
.backgroundColor(0xaabbcc)
}
.size(width: 100, height: 50)
Badge(BadgeParams(
count: 110,
style: BadgeStyle(color: Color(0xFFFFFF), fontSize: 16, badgeSize: 20, badgeColor: Color.RED),
position: BadgePosition.RightTop,
maxCount: 99)
) {
Text("Badge")
.size(width: 100, height: 50)
.fontSize(20)
.backgroundColor(0xaabbcc)
}
.size(width: 100, height: 50)
}
}
.padding(10)
.width(100.percent)
.height(100.percent)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
样例运行结果如下图所示:
BadgeParamWithString
可以根据字符串创建提醒组件,各参数说明如下:
- value:提示内容的文本字符串。
简单样例如下所示:
@Entry @Component struct ComponentTest {
build() {
Column() {
Row({space: 20}) {
Badge({
value: "aaa", // 设置 badge 的显示文本
position: BadgePosition.RightTop,// 设置 badge 显示在右上角
style: {badgeColor: Color.Red} // 设置 badge 的显示样式
}) {
Text("Badge")
.size({width: 100, height: 50})
.fontSize(20)
.backgroundColor("#aabbcc")
}
.size({width: 100, height: 50})
Badge({
value: "bbb", // 设置 badge 的显示文本
position: BadgePosition.RightTop,// 设置 badge 显示在右上角
style: {badgeColor: Color.Red} // 设置 badge 的显示样式
}) {
Text("Badge")
.size({width: 100, height: 50})
.fontSize(20)
.backgroundColor("#aabbcc")
}
.size({width: 100, height: 50})
}
}
.width("100%")
.height("100%")
.padding(20)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
@Entry @Component class MyView {
func build() {
Column(10) {
Row(20) {
Badge(BadgeParams(
value: "aaa",
style: BadgeStyle(color: Color(0xFFFFFF), fontSize: 16, badgeSize: 20, badgeColor: Color.RED),
position: BadgePosition.RightTop)
) {
Text("Badge")
.size(width: 100, height: 50)
.fontSize(20)
.backgroundColor(0xaabbcc)
}
.size(width: 100, height: 50)
Badge(BadgeParams(
value: "bbb",
style: BadgeStyle(color: Color(0xFFFFFF), fontSize: 16, badgeSize: 20, badgeColor: Color.RED),
position: BadgePosition.RightTop)
) {
Text("Badge")
.size(width: 100, height: 50)
.fontSize(20)
.backgroundColor(0xaabbcc)
}
.size(width: 100, height: 50)
}
}
.padding(10)
.width(100.percent)
.height(100.percent)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
样例运行结果如下图所示:
# 5.7.2:Badge 属性介绍
declare class BadgeAttribute extends CommonMethod<BadgeAttribute> {
}
1
2
2
由源码可知,Badge
没有定义额外的自有属性。
# 5.7.2:Badge 完整样例
@Entry @Component struct ComponentTest {
@State counts: number = 1
@State message: string = 'new'
build() {
Flex({ justifyContent: FlexAlign.SpaceAround }) {
Badge({
count: this.counts,
maxCount: 99,
style: { color: 0xFFFFFF, fontSize: 16, badgeSize: 20, badgeColor: Color.Red }
}) {
Button('message')
.onClick(() => {
this.counts++
})
.width(100).height(50).backgroundColor(0x317aff)
}.width(100).height(50)
Badge({
value: this.message,
style: { color: 0xFFFFFF, fontSize: 9, badgeSize: 20, badgeColor: Color.Blue }
}) {
Text('message')
.width(80)
.height(50)
.fontSize(18)
.lineHeight(37)
.borderRadius(10)
.textAlign(TextAlign.Center)
.backgroundColor(Color.Pink)
}
.width(80).height(50)
Badge({
value: ' ',
position: BadgePosition.RightTop,
style: { badgeSize: 6, badgeColor: Color.Red }
}) {
Text('message')
.width(90)
.height(50)
.fontSize(18)
.lineHeight(37)
.borderRadius(10)
.textAlign(TextAlign.Center)
.backgroundColor(Color.Pink)
}.width(90).height(50)
}.width('100%').margin({ top: 10 })
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
@Entry @Component class MyView {
@State var counts: Int32 = 1;
@State var message: String = "new";
func build() {
Flex(FlexParams(justifyContent: FlexAlign.SpaceAround)) {
Badge(BadgeParams(
count: this.counts,
style: BadgeStyle(color: Color(0xFFFFFF), fontSize: 16, badgeSize: 20, badgeColor: Color.RED),
position: BadgePosition.RightTop,
maxCount: 99)
) {
Button('message')
.onClick({ =>
this.counts++
})
.width(100).height(50).backgroundColor(0x317aff)
}.width(100).height(50)
Badge(BadgeParams(
value: this.message,
style: BadgeStyle(color: Color(0xFFFFFF), fontSize: 16, badgeSize: 20, badgeColor: Color.RED),
position: BadgePosition.RightTop)
) {
Text('message')
.width(80)
.height(50)
.fontSize(18)
.lineHeight(37)
.borderRadius(10)
.textAlign(TextAlign.Center)
.backgroundColor(0xffc0cb)
}
.width(80).height(50)
Badge(BadgeParams(
value: " ",
style: BadgeStyle(color: Color(0xFFFFFF), fontSize: 16, badgeSize: 20, badgeColor: Color.RED),
position: BadgePosition.RightTop)
) {
Text('message')
.width(90)
.height(50)
.fontSize(18)
.lineHeight(37)
.borderRadius(10)
.textAlign(TextAlign.Center)
.backgroundColor(0xffc0cb)
}.width(90).height(50)
}.width(100.percent).margin(top: 10)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
样例运行结果如下图所示:
📢:Badge 组件使用的时候要设置具体宽高,否则会铺满父组件。
(adsbygoogle = window.adsbygoogle || []).push({});
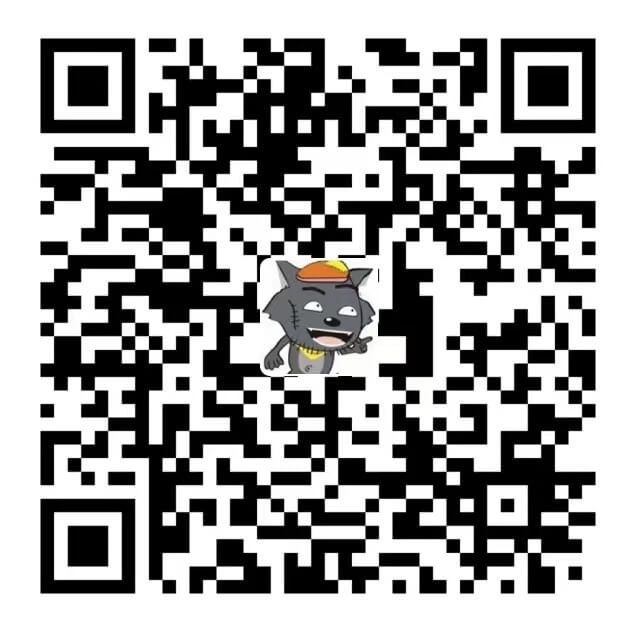
请作者喝杯咖啡
©arkui.club版权所有,禁止私自转发、克隆网站。