# 8.4:Rect、Shape
Rect
也是线性类绘制组件,它绘制时的坐标参考系是自身。左上角坐标为(0,0),右下角坐标为:(width,height)。 Shape
是绘制类组件的父组件,它定义了所有绘制组件均支持的通用属性。
# 8.4.1:Rect
Rect
用来绘制矩形组件。
# 8.4.1.1:Rect定义介绍
interface Rect extends RectAttribute<Rect> {
new (
value?: { width?: number | string, height?: number | string, radius?: number | string | Array<any> } |
{
width?: number | string, height?: number | string, radiusWidth?: number | string,
radiusHeight?: number | string
}): Rect;
(value?: {width?: number | string,height?: number | string,radius?: number | string | Array<any> } |
{
width?: number | string,height?: number | string,radiusWidth?: number | string,radiusHeight?: number | string}): Rect;
}
1
2
3
4
5
6
7
8
9
10
11
2
3
4
5
6
7
8
9
10
11
- width:绘制矩形的宽度。
- height:绘制矩形的高度。
- radius:圆角半径,支持分别设置四个角的圆角度数。
- radiusWidth:圆角宽度。
- radiusHeight:圆角高度。
# 8.4.1.2:Rect定义介绍
declare class RectAttribute extends CommonShapeMethod<RectAttribute> {
radius(value: number | string | Array<any>): RectAttribute;
radiusWidth(value: number | string): RectAttribute;
radiusHeight(value: number | string): RectAttribute;
}
1
2
3
4
5
2
3
4
5
- radius:圆角半径,支持分别设置四个角的圆角度数。
- radiusWidth:圆角宽度。
- radiusHeight:圆角高度。
简单样例如下所示:
@Entry @Component struct Index {
build() {
Column({ space: 10 }) {
Rect() {
}
.width(220)
.height(50)
.fill(Color.Pink)
Rect() {
}
.width(220)
.height(50)
.radius(10)
.fill(Color.Pink)
Rect() {
}
.width(220)
.height(50)
.radiusWidth(25)
.radiusHeight(25)
.fill(Color.Pink)
Rect() {
}
.width(220)
.height(50)
.radiusWidth(25)
.fill(Color.Pink)
.strokeWidth(3)
.stroke("#ff0000")
Rect() {
}
.width(220)
.height(50)
.radiusWidth(25)
.fill(Color.Pink)
.strokeWidth(3)
.stroke("#ff0000")
.strokeDashArray([10])
}
.padding(10)
.size({ width: "100%", height: '100%' })
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
样例运行结果如下图所示:
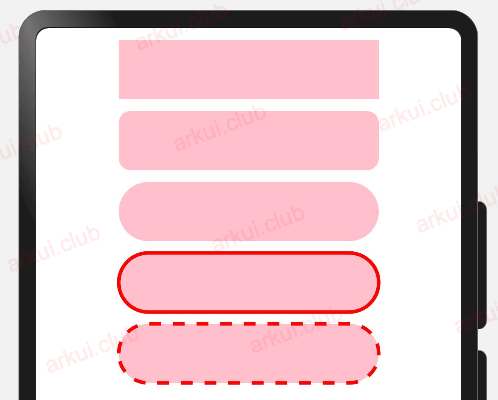
# 8.4.2:Shape
绘制组件的父组件,父组件中会描述所有绘制组件均支持的通用属性。
1、绘制组件使用 Shape
作为父组件,实现类似 SVG 的效果。
2、绘制组件单独使用,用于在页面上绘制指定的图形。
# 8.4.2.1:Shape定义介绍
interface ShapeInterface {
new (value?: PixelMap): ShapeAttribute;
(value?: PixelMap): ShapeAttribute;
}
1
2
3
4
2
3
4
- value:设置填充内容,可以接收一个
PixelMap
。
# 8.4.2.2:Shape属性介绍
declare class ShapeAttribute extends CommonMethod<ShapeAttribute> {
viewPort(value: { x?: number | string; y?: number | string; width?: number | string; height?: number | string }): ShapeAttribute;
stroke(value: ResourceColor): ShapeAttribute;
fill(value: ResourceColor): ShapeAttribute;
strokeDashOffset(value: number | string): ShapeAttribute;
strokeDashArray(value: Array<any>): ShapeAttribute;
strokeLineCap(value: LineCapStyle): ShapeAttribute;
strokeLineJoin(value: LineJoinStyle): ShapeAttribute;
strokeMiterLimit(value: number | string): ShapeAttribute;
strokeOpacity(value: number | string | Resource): ShapeAttribute;
fillOpacity(value: number | string | Resource): ShapeAttribute;
strokeWidth(value: number | string): ShapeAttribute;
antiAlias(value: boolean): ShapeAttribute;
mesh(value: Array<any>, column: number, row: number): ShapeAttribute;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
2
3
4
5
6
7
8
9
10
11
12
13
14
15
- viewPort:设置 Shape 的视图窗口。
- stroke:设置 Shape 的边框颜色。
- fill:设置 Shape 的填充颜色。
- strokeDashArray:设置 Shape 的边框间距。
- strokeDashOffset:设置 Shape 的边框绘制起点的偏移量。
- strokeLineCap:设置 Shape 的边框路径端点绘制样式。
- strokeLineJoin:设置 Shape 的边框拐角绘制样式。
- strokeMiterLimit:设置 Shape 的边框锐角绘制成斜角的极限值。
- strokeOpacity:设置 Shape 的边框不透明度。
- strokeWidth:设置 Shape 的边框宽度。
- antiAlias:设置 Shape 是否开启抗锯齿。默认开启。
简单样例如下所示:
@Entry @Component struct Index {
build() {
Column({ space: 10 }) {
Shape() {
Rect()
.width(300)
.height(50)
Ellipse()
.width(300)
.height(50)
.offset({ x: 0, y: 60 })
Path()
.width(300)
.height(10)
.commands('M0 0 L900 0')
.offset({ x: 0, y: 120 })
}
.viewPort({ x: -2, y: -2, width: 304, height: 130 })
.fill(0x317Af7)
.stroke(Color.Black)
.strokeWidth(4)
.strokeDashArray([20])
.strokeDashOffset(10)
.strokeLineCap(LineCapStyle.Round)
.strokeLineJoin(LineJoinStyle.Round)
.antiAlias(true)
Shape() {
Rect()
.width(300)
.height(50)
}
.viewPort({ x: -1, y: -1, width: 302, height: 52 })
.fill(0x317Af7)
.stroke(Color.Black)
.strokeWidth(2)
Shape() {
Path()
.width(300)
.height(10)
.commands('M0 0 L900 0')
}
.viewPort({ x: 0, y: -5, width: 300, height: 10 })
.stroke(0xEE8443)
.strokeWidth(20)
.strokeDashArray([4])
Shape() {
Path()
.width(300)
.height(10)
.commands('M0 0 L900 0')
}
.viewPort({ x: 0, y: 0, width: 300, height: 20 })
.stroke(0xEE8443)
.strokeWidth(10)
.strokeOpacity(0.5)
Shape() {
Path()
.width(300)
.height(10)
.commands('M0 0 L900 0')
}
.viewPort({ x: -5, y: -5, width: 300, height: 10 })
.stroke(0xEE8443)
.strokeWidth(10)
.strokeDashArray([20])
.strokeLineCap(LineCapStyle.Round)
Shape() {
Rect()
.width(300)
.height(100)
}
.viewPort({ x: -5, y: -5, width: 310, height: 120 })
.fill(0x317Af7)
.stroke(0xEE8443)
.strokeWidth(10)
.strokeLineJoin(LineJoinStyle.Round)
Shape() {
Path()
.width(300)
.height(60)
.commands('M0 0 L400 0 L400 200 Z')
}
.viewPort({ x: -80, y: -5, width: 310, height: 100 })
.fill(0x317Af7)
.stroke(0xEE8443)
.strokeWidth(10)
.strokeLineJoin(LineJoinStyle.Miter)
.strokeMiterLimit(10)
}
.padding(10)
.size({ width: "100%", height: '100%' })
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
样例运行结果如下图所示:
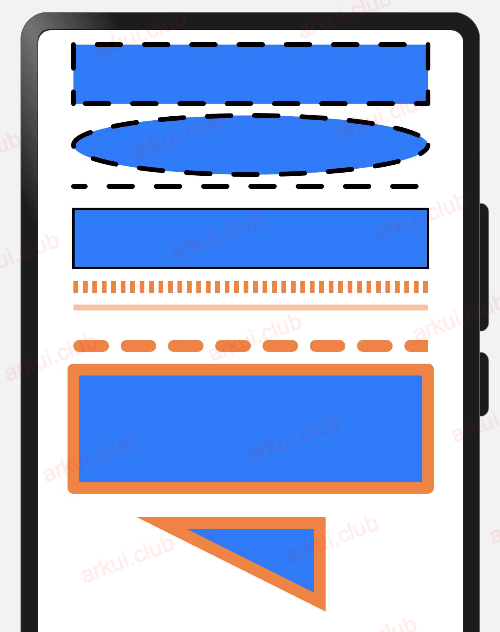
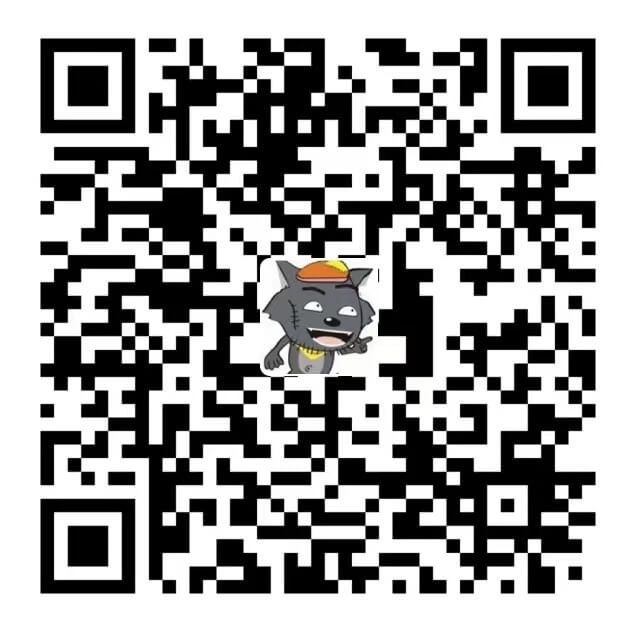
请作者喝杯咖啡
©arkui.club版权所有,禁止私自转发、克隆网站。