# 18.7:键盘操作
APP 开发过程中可能会有隐藏键盘的操作,比如用户输入完毕后可以自动隐藏键盘,ArkUI 开发框架在 @ohos.inputmethod
模块里提供了对键盘显示和隐藏的操作方法,本节笔者简单介绍一下它们的基本使用。
# 18.7.1:显示键盘
对键盘操作先要引入@ohos.inputmethod
模块,然后调用相关 API 即可,伪代码如下:
// 引入模块
import inputMethod from '@ohos.inputmethod';
// 显示键盘
private showKeyboard() {
inputMethod.getController().showSoftKeyboard().then(() => {
console.log("show keyboard success")
}).catch((error) => {
console.log("show keyboard failure: " + error)
})
}
1
2
3
4
5
6
7
8
9
10
11
2
3
4
5
6
7
8
9
10
11
# 18.7.2:隐藏键盘
对键盘操作先要引入@ohos.inputmethod
模块,然后调用相关 API 即可,伪代码如下:
// 引入模块
import inputMethod from '@ohos.inputmethod';
// 隐藏键盘
private hideKeyboard() {
inputMethod.getController().hideSoftKeyboard().then(() => {
console.log("hide keyboard success")
}).catch((error) => {
console.log("hide keyboard failure: " + error)
})
}
1
2
3
4
5
6
7
8
9
10
11
2
3
4
5
6
7
8
9
10
11
# 18.7.3:监听键盘
ArkUI 开发框架在 '@ohos.window'
模块里提供了对键盘高度变换的监听,伪代码如下:
// 引入模块
import window from '@ohos.window';
// 监听键盘高度变化
private monitorKeyboardHeightChanged() {
let context = getContext(this) as Context
window.getLastWindow(context).then((lastWindow) => {
lastWindow.on("keyboardHeightChange", (height) => {
console.log("keyboardHeightChanged: " + height)
})
}).catch((error) => {
console.log("getLastWindow error: " + error)
})
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
2
3
4
5
6
7
8
9
10
11
12
13
14
# 18.7.4:完整样例
import inputMethod from '@ohos.inputmethod';
import window from '@ohos.window';
@Entry @Component struct ArkUIClubTest {
build() {
Column({space: 10}) {
Button("显示键盘")
.onClick(() => {
this.showKeyboard()
})
Button("隐藏键盘")
.onClick(() => {
this.hideKeyboard()
})
Button("监听键盘高度变化")
.onClick(() => {
this.monitorKeyboardHeightChanged()
})
Button("取消监听键盘高度变化")
.onClick(() => {
this.unMonitorKeyboardHeightChanged()
})
}
.width("100%")
.height("100%")
.backgroundColor("#aabbcc")
.padding(10)
}
private showKeyboard() {
inputMethod.getController().showSoftKeyboard().then(() => {
console.log("show keyboard success")
}).catch((error) => {
console.log("show keyboard failure: " + error)
})
}
private hideKeyboard() {
inputMethod.getController().hideSoftKeyboard().then(() => {
console.log("hide keyboard success")
}).catch((error) => {
console.log("hide keyboard failure: " + error)
})
}
private monitorKeyboardHeightChanged() {
let context = getContext(this) as Context
window.getLastWindow(context).then((lastWindow) => {
lastWindow.on("keyboardHeightChange", (height) => {
console.log("keyboardHeightChanged: " + height)
})
}).catch((error) => {
console.log("getLastWindow error: " + error)
})
}
private unMonitorKeyboardHeightChanged() {
let context = getContext(this) as Context
window.getLastWindow(context).then((lastWindow) => {
lastWindow.off("keyboardHeightChange")
})
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
样例运行结果如下图所示:
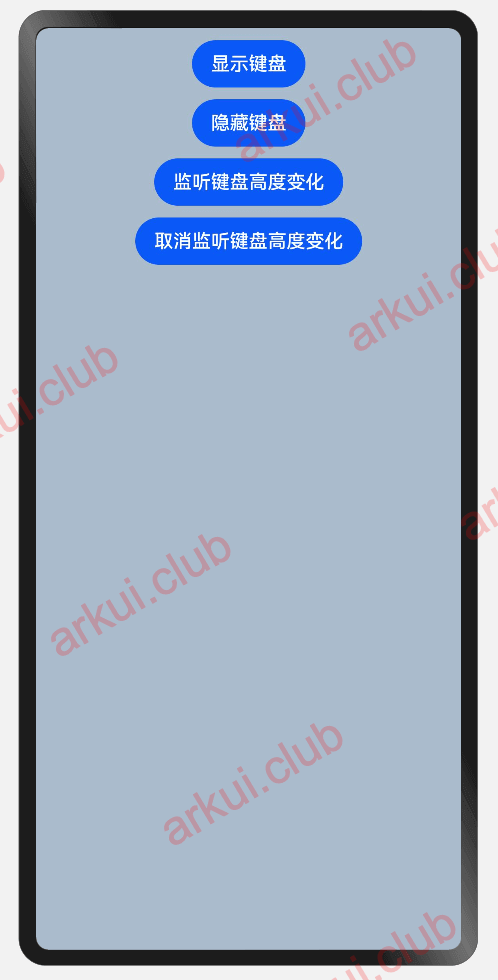
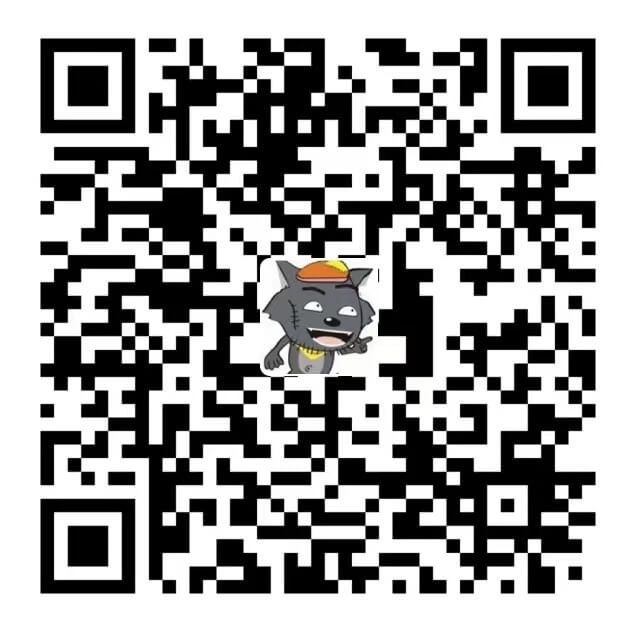
请作者喝杯咖啡
©arkui.club版权所有,禁止私自转发、克隆网站。