# 3.5:对齐设置
# 3.5.1:对齐方式
declare class CommonMethod<T> {
align(value: Alignment): T;
}
1
2
3
2
3
设置元素内容的对齐方式,只有当设置的 width
和 height
大小超过元素本身内容大小时生效。
简单样例如下所示:
@Entry @Component struct Index {
build() {
Column({ space: 10 }) {
Text("align") // 默认样式
.fontSize(20)
.backgroundColor((Color.Pink))
Text("align") // 组件尺寸默认等于内容尺寸
.fontSize(20)
.align(Alignment.TopStart) // 组件尺寸默认等于内容尺寸,不符合条件
.backgroundColor((Color.Pink))
Text("align")
.fontSize(20)
.size({width: 200, height: 60})// 组件尺寸大于内容尺寸,符合条件
.align(Alignment.TopStart) // 设置内容对齐方式
.backgroundColor((Color.Pink))
}
.width('100%')
.height("100%")
.padding(10)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
@Entry @Component class MyView {
func build() {
Column(10) {
Text("align") // 默认样式
.fontSize(20)
.backgroundColor((0xffc0cb))
Text("align") // 组件尺寸默认等于内容尺寸
.fontSize(20)
.align(Alignment.TopStart) // 组件尺寸默认等于内容尺寸,不符合条件
.backgroundColor((0xffc0cb))
Text("align")
.fontSize(20)
.size(width: 200, height: 60) // 组件尺寸大于内容尺寸,符合条件
.align(Alignment.TopStart) // 设置内容对齐方式
.backgroundColor((0xffc0cb))
}
.width(100.percent)
.height(100.percent)
.padding(10)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
样例运行结果如下所示:
# 3.5.2:布局方向
declare class CommonMethod<T> {
direction(value: Direction): T;
}
declare enum Direction {
Ltr,
Rtl,
Auto
}
1
2
3
4
5
6
7
8
9
2
3
4
5
6
7
8
9
设置子组件在水平方向上的布局方式,Direction
定义了一下 3 种布局方式:
- Ltr:元素从左到右布局。
- Rtl:元素从右到左布局。
- Auto(默认值):使用系统默认布局方向。
简单样例如下所示:
@Entry @Component struct Index {
build() {
Column({ space: 10 }) {
Row({space: 10}) { // 不设置子组件的对齐方式时采用默认值
Text('1')
.height(50)
.width('25%')
.fontSize(16)
.backgroundColor("#aabbcc")
Text('2')
.height(50)
.width('25%')
.fontSize(16)
.backgroundColor("#bbccaa")
Text('3')
.height(50)
.width('25%')
.fontSize(16)
.backgroundColor("#ccaabb")
}
.width('90%')
.backgroundColor(Color.Pink)
Row({space: 10}) {
Text('1')
.height(50)
.width('25%')
.fontSize(16)
.backgroundColor("#aabbcc")
Text('2')
.height(50)
.width('25%')
.fontSize(16)
.backgroundColor("#bbccaa")
Text('3')
.height(50)
.width('25%')
.fontSize(16)
.backgroundColor("#ccaabb")
}
.width('90%')
.backgroundColor(Color.Pink)
.direction(Direction.Rtl) // 设置子组件的对齐方式为Rtl
}
.width('100%')
.height("100%")
.padding(10)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
@Entry @Component class MyView {
func build() {
Column(10) {
Row(10) { // 不设置子组件的对齐方式时采用默认值
Text('1')
.height(50)
.width(25.percent)
.fontSize(16)
.backgroundColor(0xaabbcc)
Text('2')
.height(50)
.width(25.percent)
.fontSize(16)
.backgroundColor(0xbbccaa)
Text('3')
.height(50)
.width(25.percent)
.fontSize(16)
.backgroundColor(0xccaabb)
}
.width(90.percent)
.backgroundColor(0xffc0cb)
Row(10) {
Text('1')
.height(50)
.width(25.percent)
.fontSize(16)
.backgroundColor(0xaabbcc)
Text('2')
.height(50)
.width(25.percent)
.fontSize(16)
.backgroundColor(0xbbccaa)
Text('3')
.height(50)
.width(25.percent)
.fontSize(16)
.backgroundColor(0xccaabb)
}
.width(90.percent)
.backgroundColor(0xffc0cb)
.direction(Direction.Rtl) // 设置子组件的对齐方式为Rtl
}
.width(100.percent)
.height(100.percent)
.padding(10)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
样例运行结果如下图所示:
# 3.5.3:约束条件
declare class CommonMethod<T> {
alignRules(value: AlignRuleOption): T;
}
// 约束规则
declare interface AlignRuleOption {
left?: { anchor: string, align: HorizontalAlign };
right?: { anchor: string, align: HorizontalAlign };
middle?: { anchor: string, align: HorizontalAlign };
top?: { anchor: string, align: VerticalAlign };
bottom?: { anchor: string, align: VerticalAlign };
center?: { anchor: string, align: VerticalAlign };
}
1
2
3
4
5
6
7
8
9
10
11
12
13
2
3
4
5
6
7
8
9
10
11
12
13
设置子组件在父组件 RelativeContainer
中的对齐方式,分为水平对齐规则和竖直对齐规则,分别说明如下:
- 水平对齐规则
- left: 设置左对齐参数。
- middle: 设置中间对齐的参数。
- right: 设置右对齐参数。
- 竖直对齐规则
- top: 设置顶部对齐的参数。
- bottom: 设置底部对齐的参数。
- center: 设置中心对齐的参数。
简单样例如下所示:
@Entry @Component struct Index {
build() {
Column() {
Row() {
RelativeContainer() {
Row()
.width(100)
.height(100)
.backgroundColor("#FF3333")
.alignRules({
top: {
anchor: "__container__",
align: VerticalAlign.Top
},
left: {
anchor: "__container__",
align: HorizontalAlign.Start
}
})
.id("row1")
Row()
.width(100)
.height(100)
.backgroundColor("#FFCC00")
.alignRules({
top: {
anchor: "__container__",
align: VerticalAlign.Top
},
right: {
anchor: "__container__",
align: HorizontalAlign.End
}
})
.id("row2")
Row().height(100)
.backgroundColor("#FF6633")
.alignRules({
top: {
anchor: "row1",
align: VerticalAlign.Bottom
},
left: {
anchor: "row1",
align: HorizontalAlign.End
},
right: {
anchor: "row2",
align: HorizontalAlign.Start
}
})
.id("row3")
Row()
.backgroundColor("#FF9966")
.alignRules({
top: {
anchor: "row3",
align: VerticalAlign.Bottom
},
bottom: {
anchor: "__container__",
align: VerticalAlign.Bottom
},
left: {
anchor: "__container__",
align: HorizontalAlign.Start
},
right: {
anchor: "row1",
align: HorizontalAlign.End
}
})
.id("row4")
Row()
.backgroundColor("#FF66FF")
.alignRules({
top: {
anchor: "row3",
align: VerticalAlign.Bottom
},
bottom: {
anchor: "__container__",
align: VerticalAlign.Bottom
},
left: {
anchor: "row2",
align: HorizontalAlign.Start
},
right: {
anchor: "__container__",
align: HorizontalAlign.End
}
})
.id("row5")
}
.width(300)
.height(300)
.border({ width: 3, color: "#6699FF" })
}
}
.width("100%")
.height("100%")
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
@Entry @Component class MyView {
func build() {
Row() {
RelativeContainer() {
Row().width(100).height(100).backgroundColor(0xff3333).alignRules(
AlignRuleOption(
top: VerticalAnchor("__container__", VerticalAlign.Top),
left: HorizontalAnchor("__container__", HorizontalAlign.Start)
)
).id("row1")
Row().width(100).height(100).backgroundColor(0xFFCC00).alignRules(
AlignRuleOption(
top: VerticalAnchor("__container__", VerticalAlign.Top),
right: HorizontalAnchor("__container__", HorizontalAlign.End)
)
).id("row2")
Row().height(100).backgroundColor(0xFF6633).alignRules(
AlignRuleOption(
top: VerticalAnchor("row1", VerticalAlign.Bottom),
left: HorizontalAnchor("row1", HorizontalAlign.End),
right: HorizontalAnchor("row2", HorizontalAlign.Start)
)
).id("row3")
Row().backgroundColor(0xFF9966).alignRules(
AlignRuleOption(
top: VerticalAnchor("row3", VerticalAlign.Bottom),
bottom: VerticalAnchor("__container__", VerticalAlign.Bottom),
left: HorizontalAnchor("__container__", HorizontalAlign.Start),
right: HorizontalAnchor("row1", HorizontalAlign.End)
)
).id("row4")
Row().backgroundColor(0xff3333).alignRules(
AlignRuleOption(
top: VerticalAnchor("row3", VerticalAlign.Bottom),
bottom: VerticalAnchor("__container__", VerticalAlign.Bottom),
left: HorizontalAnchor("row2", HorizontalAlign.Start),
right: HorizontalAnchor("__container__", HorizontalAlign.End)
)
).id("row5")
}.width(300).height(300).margin(left: 50.vp).border(width: 2.vp, color: Color(0x6699ff))
}.height(100.percent)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
样例运行结果如下图所示:
📢:有关约束规则的使用详见第 5 章 第 9 节 的介绍。
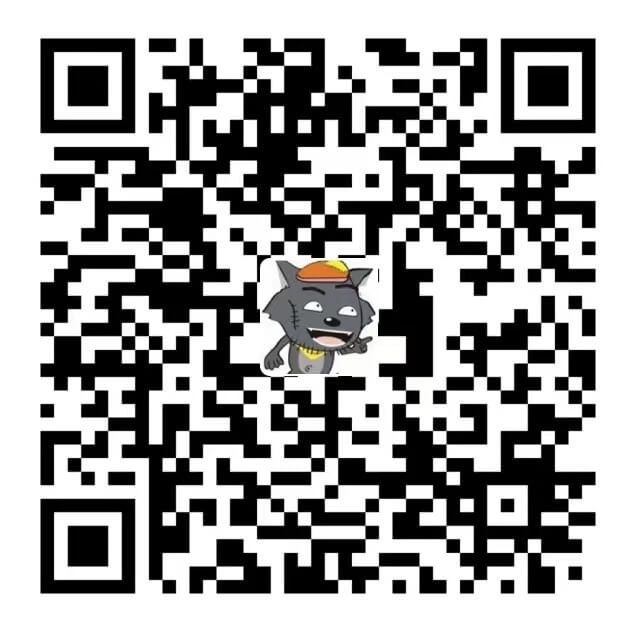
请作者喝杯咖啡
©arkui.club版权所有,禁止私自转发、克隆网站。