3.6:背景设置
3.6.1:背景色设置
export declare class CommonMethod<T> {
backgroundColor(value: ResourceColor): T;
}
declare type ResourceColor = Color | number | string | Resource;
1
2
3
4
5
设置组件的背景颜色, ResourceColor
类型支持 Color
| number
| string
| Resource
四种。
简单样例如下图所示:
@Entry @Component struct Index {
build() {
Column() {
Row() {
Text()
.height(30)
.backgroundColor("#aabbcc")
.layoutWeight(1)
Text()
.height(30)
.backgroundColor("#aaccbb")
.layoutWeight(1)
Text()
.height(30)
.backgroundColor(Color.Pink)
.layoutWeight(1)
}
.padding(10)
.width("100%")
}
.width("100%")
.height("100%")
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
@Entry @Component class MyView {
func build() {
Column() {
Row() {
Text("")
.height(30)
.backgroundColor(0xaabbcc)
.layoutWeight(1)
Text("")
.height(30)
.backgroundColor(0xaaccbb)
.layoutWeight(1)
Text("")
.height(30)
.backgroundColor(0xffc0cb)
.layoutWeight(1)
}
.padding(10)
.width(100.percent)
}
.width(100.percent)
.height(100.percent)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
样例运行结果如下图所示:
3.6.2:背景图设置
export declare class CommonMethod<T> {
backgroundImage(src: ResourceStr, repeat?: ImageRepeat): T;
}
1
2
3
设置组件的背景图片,repeat
参数可以设置图片的填充模式,简单样例如下所示:
Text("图片背景图")
.fontSize(30)
.fontColor(Color.Red)
.size({width: 220, height: 90})
.backgroundImage($r('app.media.test'))
1
2
3
4
5
@Entry @Component class MyView {
func build() {
Column() {
Text("图片背景图")
.fontSize(30)
.fontColor(0xff0000)
.size(width: 220, height: 90)
.backgroundImage(src: @r(app.media.test))
}
.width(100.percent)
.height(100.percent)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
样例运行结果如下图所示:
3.6.3:边框设置
export declare class CommonMethod<T> {
border(value: BorderOptions): T;
borderStyle(value: BorderStyle): T;
borderWidth(value: Length): T;
borderColor(value: ResourceColor): T;
borderRadius(value: Length): T;
}
1
2
3
4
5
6
7
设置组件的边框样式,支持设置边框颜色、边框粗细、边框圆角以及边框的展示样式。同时设置 border
和 borderXXX
,以最后设置的值为准。
简单样例如下图所示:
@Entry @Component struct Index {
build() {
Column() {
Row({space: 10}) {
Text()
.height(70)
.layoutWeight(1)
.border({
color: Color.Red,
width: 4,
radius: 0,
style: BorderStyle.Solid
})
Text()
.height(70)
.layoutWeight(1)
.borderWidth(4)
.borderColor(Color.Red)
.borderRadius(10)
.borderStyle(BorderStyle.Dotted)
Text()
.height(70)
.layoutWeight(1)
.borderWidth(4)
.borderColor(Color.Red)
.borderRadius(10)
.borderStyle(BorderStyle.Dashed)
}
.padding(10)
.width("100%")
}
.width("100%")
.height("100%")
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
@Entry @Component class MyView {
func build() {
Column() {
Row(10) {
Text("Solid")
.height(70)
.layoutWeight(1)
.border(
color: Color(0xff0000),
width: 4.vp,
radius: 0.vp,
style: BorderStyle.Solid
)
Text("Dotted")
.height(70)
.layoutWeight(1)
.borderWidth(4)
.borderColor(0xff0000)
.borderRadius(10)
.borderStyle(BorderStyle.Dotted)
Text("Dashed")
.height(70)
.layoutWeight(1)
.borderWidth(4)
.borderColor(0xff0000)
.borderRadius(10)
.borderStyle(BorderStyle.Dashed)
}
.padding(10)
.width(100.percent)
}
.width(100.percent)
.height(100.percent)
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
样例运行结果如下图所示:
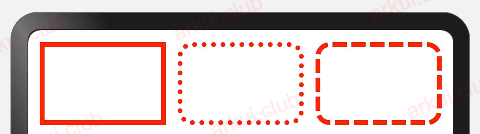