# 3.10:事件设置
# 3.10.1:点击事件
export declare class CommonMethod<T> {
onClick(event: (event?: ClickEvent) => void): T;
}
1
2
3
2
3
- onClick:给组件添加点击事件的回调,设置该回调后,当点击组件时会触发该回调。回调参数
event
包含了点击信息,比如点击坐标等。
简单样例如下所示:
点击 Text
组件,控制台会打印 text clicked AAA
的日志。
# 3.10.2:触摸事件
export declare class CommonMethod<T> {
onTouch(event: (event?: TouchEvent) => void): T;
}
1
2
3
2
3
- onTouch:给组件设置触摸事件的回调,设置该回调后,当触摸组件时会触发该回调。回调参数
event
包含了事件类型、点击坐标等信息。
简单样例如下所示:
触摸该 Text
组件,控制台会打印如下日志。
[phone][Console DEBUG] 03/15 00:05:47 88489984 app Log: touch down
[phone][Console DEBUG] 03/15 00:05:47 88489984 app Log: touch move
[phone][Console DEBUG] 03/15 00:05:47 88489984 app Log: touch move
[phone][Console DEBUG] 03/15 00:05:48 88489984 app Log: touch move
[phone][Console DEBUG] 03/15 00:05:48 88489984 app Log: touch up
1
2
3
4
5
2
3
4
5
笔者使用触摸事件实现的通用下拉刷新和上拉加载更多的 RefreshLayout
组件,部分代码如下所示:
Column() {
this.headLayout()
Column() {
this.childLayout()
}
.width(this.layoutWidth)
.height(this.layoutHeight)
.backgroundColor(Color.Pink)
.position({
x: 0,
y: this.offsetY + this.headHeight
})
this.footLayout()
}
.width(this.layoutWidth)
.height(this.layoutHeight)
.backgroundColor("#aabbcc")
.touchable(this.touchable)
.onTouch((event) => { // 添加触摸事件的回调
switch (event.type) {
case TouchType.Down:
this.onTouchDown(event); // 手指按下的事件处理
break;
case TouchType.Move:
this.onTouchMove(event); // 手指移动的事件处理
break;
case TouchType.Up: // 手指抬起的事件处理
case TouchType.Cancel:
this.onTouchUp(event);
break;
}
})
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
样例运行结果如下图所示:
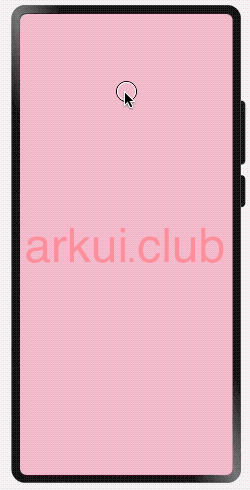
# 3.10.3:拖拽事件
export declare class CommonMethod<T> {
onDragStart(event: (event?: DragEvent, extraParams?: string) => CustomBuilder | DragItemInfo): T;
onDragEnter(event: (event?: DragEvent, extraParams?: string) => void): T;
onDragMove(event: (event?: DragEvent, extraParams?: string) => void): T;
onDragLeave(event: (event?: DragEvent, extraParams?: string) => void): T;
onDrop(event: (event?: DragEvent, extraParams?: string) => void): T;
}
1
2
3
4
5
6
7
2
3
4
5
6
7
给组件设置拖拽事件的回调,设置该回调后,当拖拽组件动作发生时会触发相应的拖拽回调,各拖拽方法说明如下:
onDragStart:第一次拖拽此事件绑定的组件时,触发回调。参数
event
包含了拖拽坐标等信息,extraParams
拖拽事件的额外信息。📢拖拽事件注意事项:
长按150毫秒(ms)可触发拖拽事件。
优先级:长按手势配置时间小于等于150毫秒时,长按手势优先触发,否则拖拽事件优先触发。
onDragEnter:拖拽进入组件范围内时,触发回调。参数
event
包含了拖拽坐标等信息,extraParams
拖拽事件的额外信息。📢拖拽事件注意事项:
- 当监听了
onDrop
事件时,此事件才有效。
- 当监听了
onDragMove:拖拽在组件范围内移动时,触发回调。参数
event
包含了拖拽坐标等信息,extraParams
拖拽事件的额外信息。📢拖拽事件注意事项:
- 当监听了
onDrop
事件时,此事件才有效。
- 当监听了
onDragLeave:拖拽离开组件范围内时,触发回调。参数
event
包含了拖拽坐标等信息,extraParams
拖拽事件的额外信息。📢拖拽事件注意事项:
- 当监听了
onDrop
事件时,此事件才有效。
- 当监听了
onDrop:绑定此事件的组件可作为拖拽释放目标,当在本组件范围内停止拖拽行为时,触发回调。参数
event
包含了拖拽坐标等信息,extraParams
拖拽事件的额外信息。
简单样例如下所示:
class DragParams {
public insertIndex: number = -1
public selectedIndex: number = -1
}
@Entry @Component struct Index {
@State numbers: string[] = ['one', 'two', 'three', 'four', 'five', 'six']
@State text: string = ''
@State bool: boolean = false
@State bool1: boolean = false
@State appleVisible: Visibility = Visibility.Visible
@State orangeVisible: Visibility = Visibility.Visible
@State bananaVisible: Visibility = Visibility.Visible
@State select: number = 0
@State currentIndex: number = 0
@Builder pixelMapBuilder() {
Column() {
Text(this.text)
.width('50%').height(60).fontSize(16).borderRadius(10)
.textAlign(TextAlign.Center).backgroundColor(Color.Yellow)
}
}
build() {
Column() {
Text('There are three Text elements here')
.fontSize(12).fontColor(0xCCCCCC).width('90%')
.textAlign(TextAlign.Start).margin(5)
Flex({ direction: FlexDirection.Row, alignItems: ItemAlign.Center, justifyContent: FlexAlign.SpaceAround }) {
Text('apple').width('25%').height(35).fontSize(16)
.textAlign(TextAlign.Center).backgroundColor(0xAFEEEE)
.visibility(this.appleVisible)
.onDragStart(() => {
this.bool = true
this.text = 'apple'
this.appleVisible = Visibility.Hidden
return this.pixelMapBuilder
})
Text('orange').width('25%').height(35).fontSize(16)
.textAlign(TextAlign.Center).backgroundColor(0xAFEEEE)
.visibility(this.orangeVisible)
.onDragStart(() => {
this.bool = true
this.text = 'orange'
this.orangeVisible = Visibility.Hidden
return this.pixelMapBuilder
})
Text('banana').width('25%').height(35).fontSize(16)
.textAlign(TextAlign.Center).backgroundColor(0xAFEEEE)
.visibility(this.bananaVisible)
.onDragStart((event: DragEvent, extraParams: string) => {
console.log('Text onDragStarts, ' + extraParams)
this.bool = true
this.text = 'banana'
this.bananaVisible = Visibility.Hidden
return this.pixelMapBuilder
})
}.border({ width: 1 }).width('90%').padding({ top: 10, bottom: 10 }).margin(10)
Text('This is a List element').fontSize(12)
.fontColor(0xCCCCCC).width('90%')
.textAlign(TextAlign.Start).margin(15)
List({ space: 20, initialIndex: 0 }) {
ForEach(this.numbers, (item: string) => {
ListItem() {
Text('' + item)
.width('100%').height(80).fontSize(16).borderRadius(10)
.textAlign(TextAlign.Center).backgroundColor(0xAFEEEE)
}
.onDragStart((event: DragEvent, extraParams: string) => {
console.log('ListItem onDragStarts, ' + extraParams)
let jsonString = JSON.parse(extraParams) as DragParams
this.bool1 = true
this.text = this.numbers[jsonString.selectedIndex]
this.select = jsonString.selectedIndex
return this.pixelMapBuilder
})
}, (item: string) => item)
}
.editMode(true)
.height('50%').width('90%').border({ width: 1 })
.divider({ strokeWidth: 2, color: 0xFFFFFF, startMargin: 20, endMargin: 20 })
.onDragEnter((event: DragEvent, extraParams: string) => {
console.log('List onDragEnter, ' + extraParams)
})
.onDragMove((event: DragEvent, extraParams: string) => {
console.log('List onDragMove, ' + extraParams)
})
.onDragLeave((event: DragEvent, extraParams: string) => {
console.log('List onDragLeave, ' + extraParams)
})
.onDrop((event: DragEvent, extraParams: string) => {
let jsonString = JSON.parse(extraParams) as DragParams
if (this.bool) {
this.numbers.splice(jsonString.insertIndex, 0, this.text)
this.bool = false
} else if (this.bool1) {
this.numbers.splice(jsonString.selectedIndex, 1)
this.numbers.splice(jsonString.insertIndex, 0, this.text)
this.bool = false
this.bool1 = false
}
})
}.width('100%').height('100%').padding({ top: 20 }).margin({ top: 20 })
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
样例运行结果如下图所示:
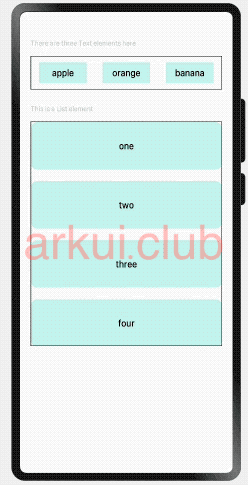
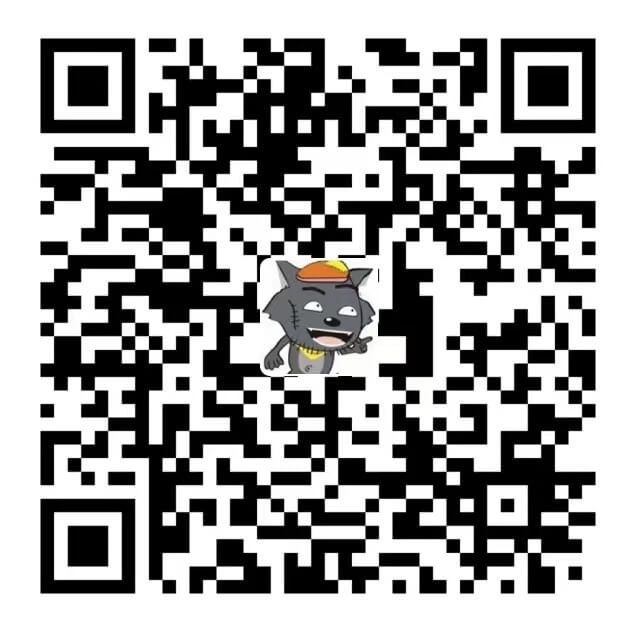
请作者喝杯咖啡
©arkui.club版权所有,禁止私自转发、克隆网站。